06. 删除 Chirps
有时,无论编辑多少次都无法修复一条消息,因此让我们让用户能够删除他们的 Chirps。
希望你现在已经开始掌握了。我们相信你会对我们添加此功能的速度印象深刻。
路由
我们将从更新我们的路由开始,以启用 chirps.destroy
路由
routes/web.php
<?php
use App\Http\Controllers\ChirpController; use App\Http\Controllers\ProfileController; use Illuminate\Support\Facades\Route; Route::get('/', function () { return view('welcome'); }); Route::get('/dashboard', function () { return view('dashboard'); })->middleware(['auth'])->name('dashboard'); Route::middleware('auth')->group(function () { Route::get('/profile', [ProfileController::class, 'edit'])->name('profile.edit'); Route::patch('/profile', [ProfileController::class, 'update'])->name('profile.update'); Route::delete('/profile', [ProfileController::class, 'destroy'])->name('profile.destroy'); }); Route::resource('chirps', ChirpController::class)- ->only(['index', 'store', 'edit', 'update'])+ ->only(['index', 'store', 'edit', 'update', 'destroy']) ->middleware(['auth', 'verified']);
require __DIR__.'/auth.php';
我们这个控制器的路由表现在如下所示
动词 | URI | 操作 | 路由名称 |
---|---|---|---|
GET | /chirps |
index | chirps.index |
POST | /chirps |
store | chirps.store |
GET | /chirps/{chirp}/edit |
edit | chirps.edit |
PUT/PATCH | /chirps/{chirp} |
update | chirps.update |
DELETE | /chirps/{chirp} |
销毁 | chirps.destroy |
更新我们的控制器
现在我们可以更新 ChirpController
类中的 destroy
方法以执行删除操作并返回到 Chirp 索引
app/Http/Controllers/ChirpController.php
<?php
namespace App\Http\Controllers; use App\Models\Chirp; use Illuminate\Http\RedirectResponse; use Illuminate\Http\Request; use Illuminate\Http\Response; use Illuminate\Support\Facades\Gate; use Illuminate\View\View; class ChirpController extends Controller {
/** * Display a listing of the resource. */ public function index(): View { return view('chirps.index', [ 'chirps' => Chirp::with('user')->latest()->get(), ]); } /** * Show the form for creating a new resource. */ public function create() { // } /** * Store a newly created resource in storage. */ public function store(Request $request): RedirectResponse { $validated = $request->validate([ 'message' => 'required|string|max:255', ]); $request->user()->chirps()->create($validated); return redirect(route('chirps.index')); } /** * Display the specified resource. */ public function show(Chirp $chirp) { // } /** * Show the form for editing the specified resource. */ public function edit(Chirp $chirp): View { Gate::authorize('update', $chirp); return view('chirps.edit', [ 'chirp' => $chirp, ]); } /** * Update the specified resource in storage. */ public function update(Request $request, Chirp $chirp): RedirectResponse { Gate::authorize('update', $chirp); $validated = $request->validate([ 'message' => 'required|string|max:255', ]); $chirp->update($validated); return redirect(route('chirps.index')); } /** * Remove the specified resource from storage. */- public function destroy(Chirp $chirp)+ public function destroy(Chirp $chirp): RedirectResponse {- //+ Gate::authorize('delete', $chirp);+ + $chirp->delete();+ + return redirect(route('chirps.index')); } }
授权
与编辑一样,我们只希望 Chirp 作者能够删除他们的 Chirp,所以让我们更新 ChirpPolicy
类中的 delete
方法
app/Policies/ChirpPolicy.php
<?php
namespace App\Policies; use App\Models\Chirp; use App\Models\User; use Illuminate\Auth\Access\HandlesAuthorization; class ChirpPolicy {
use HandlesAuthorization; /** * Determine whether the user can view any models. */ public function viewAny(User $user): bool { // } /** * Determine whether the user can view the model. */ public function view(User $user, Chirp $chirp): bool { // } /** * Determine whether the user can create models. */ public function create(User $user): bool { // } /** * Determine whether the user can update the model. */ public function update(User $user, Chirp $chirp): bool { return $chirp->user()->is($user); } /** * Determine whether the user can delete the model. */ public function delete(User $user, Chirp $chirp): bool {- //+ return $this->update($user, $chirp); }
/** * Determine whether the user can restore the model. */ public function restore(User $user, Chirp $chirp): bool { // } /** * Determine whether the user can permanently delete the model. */ public function forceDelete(User $user, Chirp $chirp): bool { // } }
与其重复 update
方法中的逻辑,我们可以通过从 destroy
方法调用 update
方法来定义相同的逻辑。任何被授权更新 Chirp 的人现在也被授权删除它。
更新我们的视图
最后,我们可以将删除按钮添加到我们之前在 chirps.index
视图中创建的下拉菜单中
resources/views/chirps/index.blade.php
<x-app-layout> <div class="max-w-2xl mx-auto p-4 sm:p-6 lg:p-8"> <form method="POST" action="{{ route('chirps.store') }}"> @csrf <textarea name="message" placeholder="{{ __('What\'s on your mind?') }}" class="block w-full border-gray-300 focus:border-indigo-300 focus:ring focus:ring-indigo-200 focus:ring-opacity-50 rounded-md shadow-sm" >{{ old('message') }}</textarea> <x-input-error :messages="$errors->get('message')" class="mt-2" /> <x-primary-button class="mt-4">{{ __('Chirp') }}</x-primary-button> </form> <div class="mt-6 bg-white shadow-sm rounded-lg divide-y"> @foreach ($chirps as $chirp) <div class="p-6 flex space-x-2"> <svg xmlns="http://www.w3.org/2000/svg" class="h-6 w-6 text-gray-600 -scale-x-100" fill="none" viewBox="0 0 24 24" stroke="currentColor" stroke-width="2"> <path stroke-linecap="round" stroke-linejoin="round" d="M8 12h.01M12 12h.01M16 12h.01M21 12c0 4.418-4.03 8-9 8a9.863 9.863 0 01-4.255-.949L3 20l1.395-3.72C3.512 15.042 3 13.574 3 12c0-4.418 4.03-8 9-8s9 3.582 9 8z" /> </svg> <div class="flex-1"> <div class="flex justify-between items-center"> <div> <span class="text-gray-800">{{ $chirp->user->name }}</span> <small class="ml-2 text-sm text-gray-600">{{ $chirp->created_at->format('j M Y, g:i a') }}</small> @unless ($chirp->created_at->eq($chirp->updated_at)) <small class="text-sm text-gray-600"> · {{ __('edited') }}</small> @endunless </div> @if ($chirp->user->is(auth()->user())) <x-dropdown> <x-slot name="trigger"> <button> <svg xmlns="http://www.w3.org/2000/svg" class="h-4 w-4 text-gray-400" viewBox="0 0 20 20" fill="currentColor"> <path d="M6 10a2 2 0 11-4 0 2 2 0 014 0zM12 10a2 2 0 11-4 0 2 2 0 014 0zM16 12a2 2 0 100-4 2 2 0 000 4z" /> </svg> </button> </x-slot> <x-slot name="content"> <x-dropdown-link :href="route('chirps.edit', $chirp)"> {{ __('Edit') }} </x-dropdown-link>+ <form method="POST" action="{{ route('chirps.destroy', $chirp) }}">+ @csrf+ @method('delete')+ <x-dropdown-link :href="route('chirps.destroy', $chirp)" onclick="event.preventDefault(); this.closest('form').submit();">+ {{ __('Delete') }}+ </x-dropdown-link>+ </form> </x-slot> </x-dropdown> @endif </div> <p class="mt-4 text-lg text-gray-900">{{ $chirp->message }}</p> </div> </div> @endforeach </div> </div> </x-app-layout>
测试一下
如果你发布了任何你不满意的内容,试试删除它!
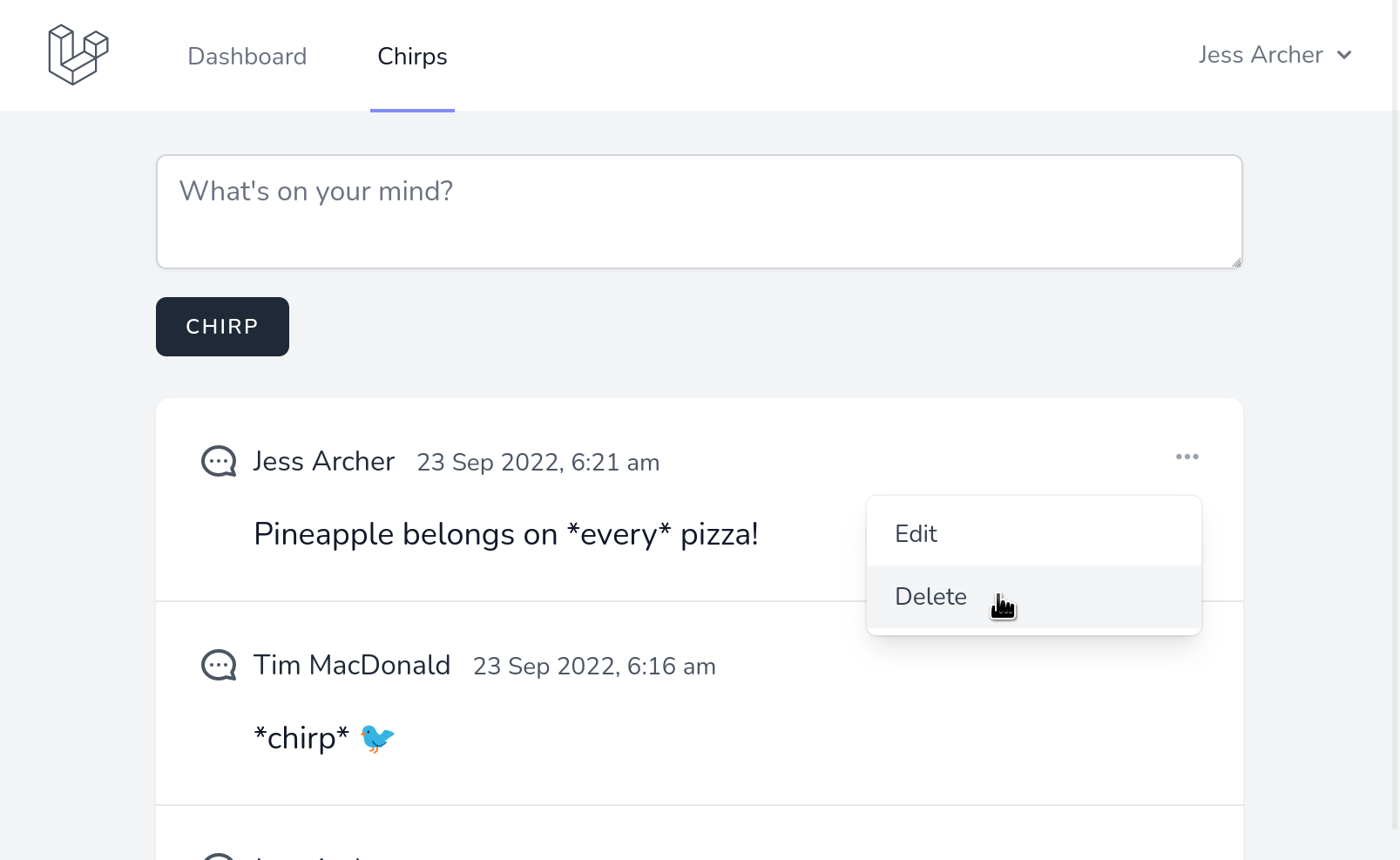